Insights
Saurabh Jain
Aug 21, 2024
What are API Keys?
API keys are security keys that guard your APIs allowing authenticated and authorized access. When building an API, you must offer keys to your users to allow them to connect to your APIs, otherwise your APIs will be public and accessible by everyone.
What do they do?
Authenticate the underlying user
Grant permissions to limit access
Limit API usage to prevent abuse
Allows revocation in case it was leaked.
Allows generating usage analytics
Limit based on number of times used
IP restrictions
and more
Implementation
Permissioning
Start by understanding and defining how you want to limit access to your system. In the following example, we create a scope called billing
and allow full CRUD access to this scope.
Next, we must attach this scope of the generated API key. Depending on your requirements you can make the scopes immutable or mutable.
Now understand how this relates to your endpoints. For endpoints, that require create
access to this scope, the middleware needs to check that the key has the required scope.
Now you must define all scopes that your system requires and then reference them in your middleware checks.
With Oneloop, you do need to write scopes and you do not need to verify scopes. Our SDKs and middleware takes care of it.
In the following image, we have defined two scopes with ease. We can add unlimited scopes as we desire.
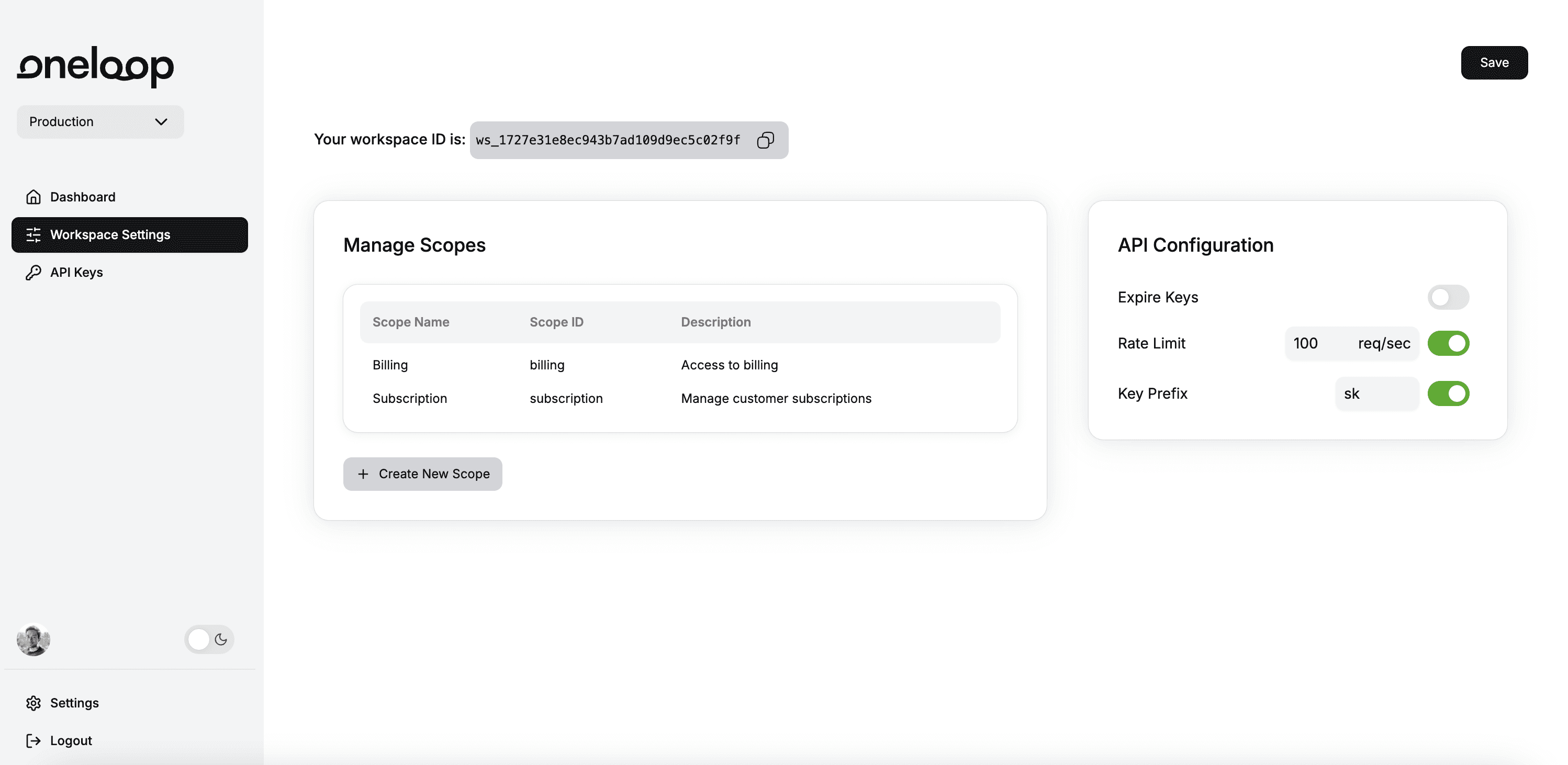
Rate Limiting
For rate-limiting you can use the fixed window algorithm. The idea is that you use an unique id and time combination to understand how many requests are made.
The hard part is the infrastructure needed and the guarantee of accuracy in a high-concurrency environment.
IP Restriction
Limit access to API from white-listed customer systems for enhanced security. For even limit access to US IPs only and so on.
Usage Limits
For trial tiers and certain billing tiers, you can limit access to your API for a limited number of times. The trick is to implement this with accuracy in a high-concurrency system where multiple requests are made at the system to different server.
Here you can toggle the switch to turn on usage limit per API Key and set it to a valid number.
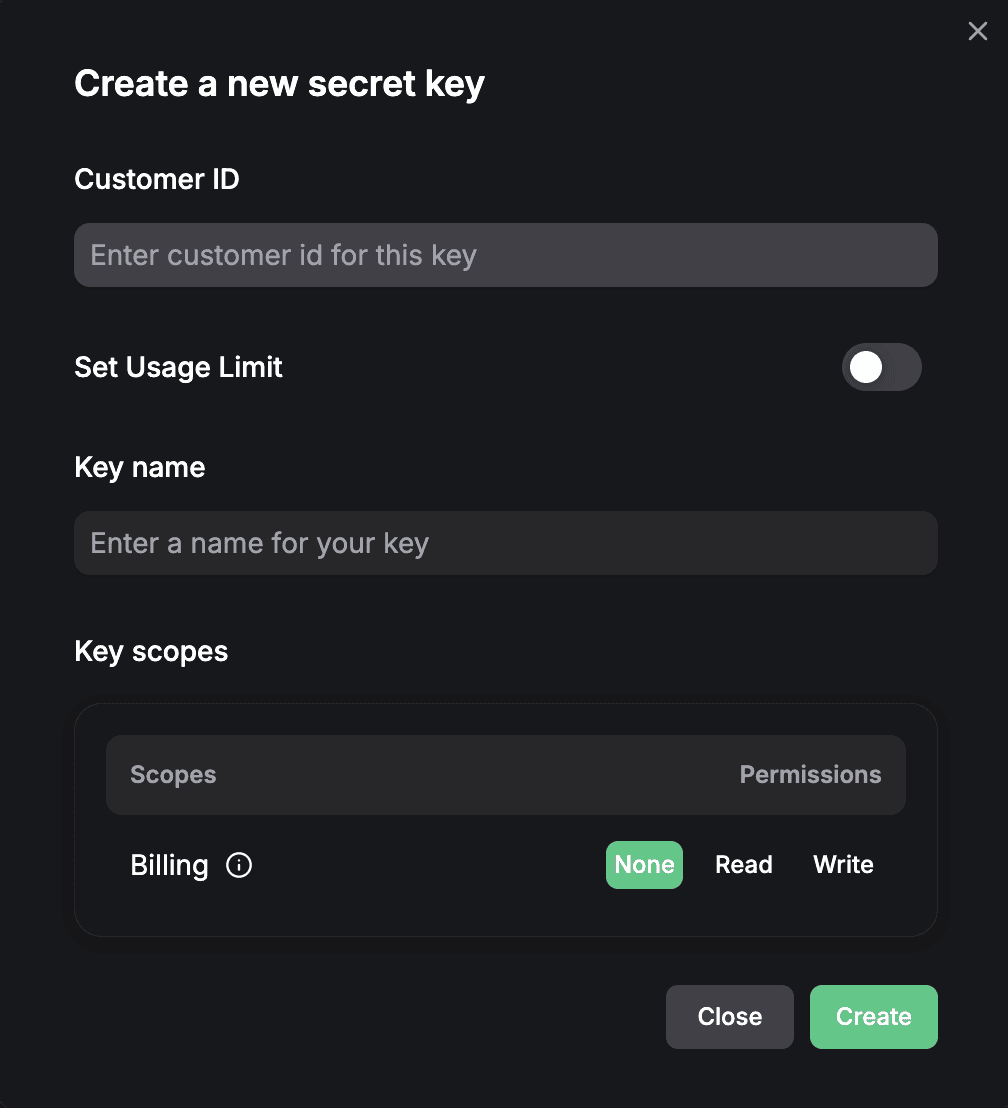
Usage Analytics
Understanding how your systems are validating keys, total verifications, workspaces with the most keys and more. This gives you insight on customer behavior and confidence in your system.
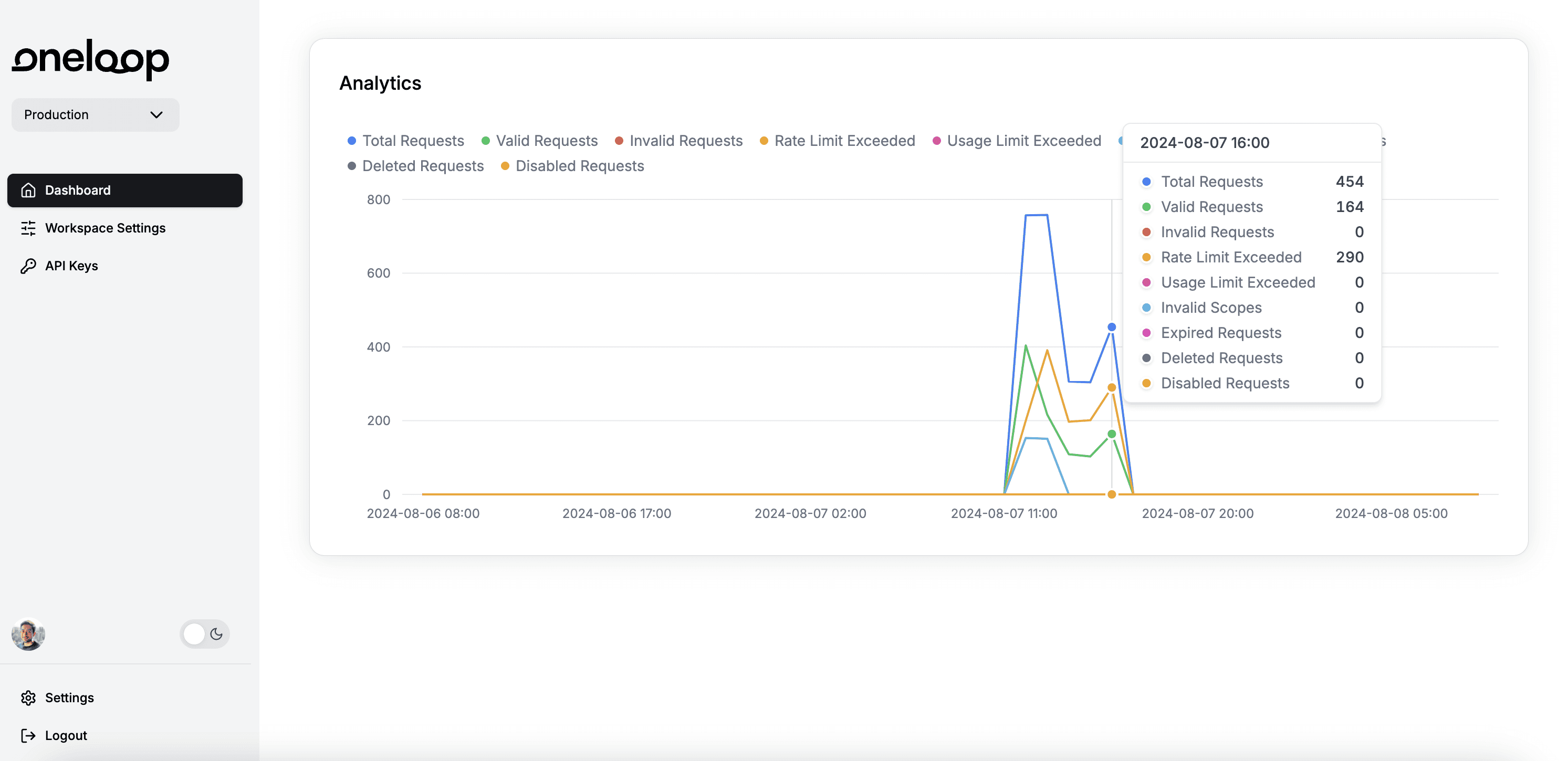
Language & SDK Support
Depending on language and/or framework you use, having pre-written middleware make verifying keys breezy.
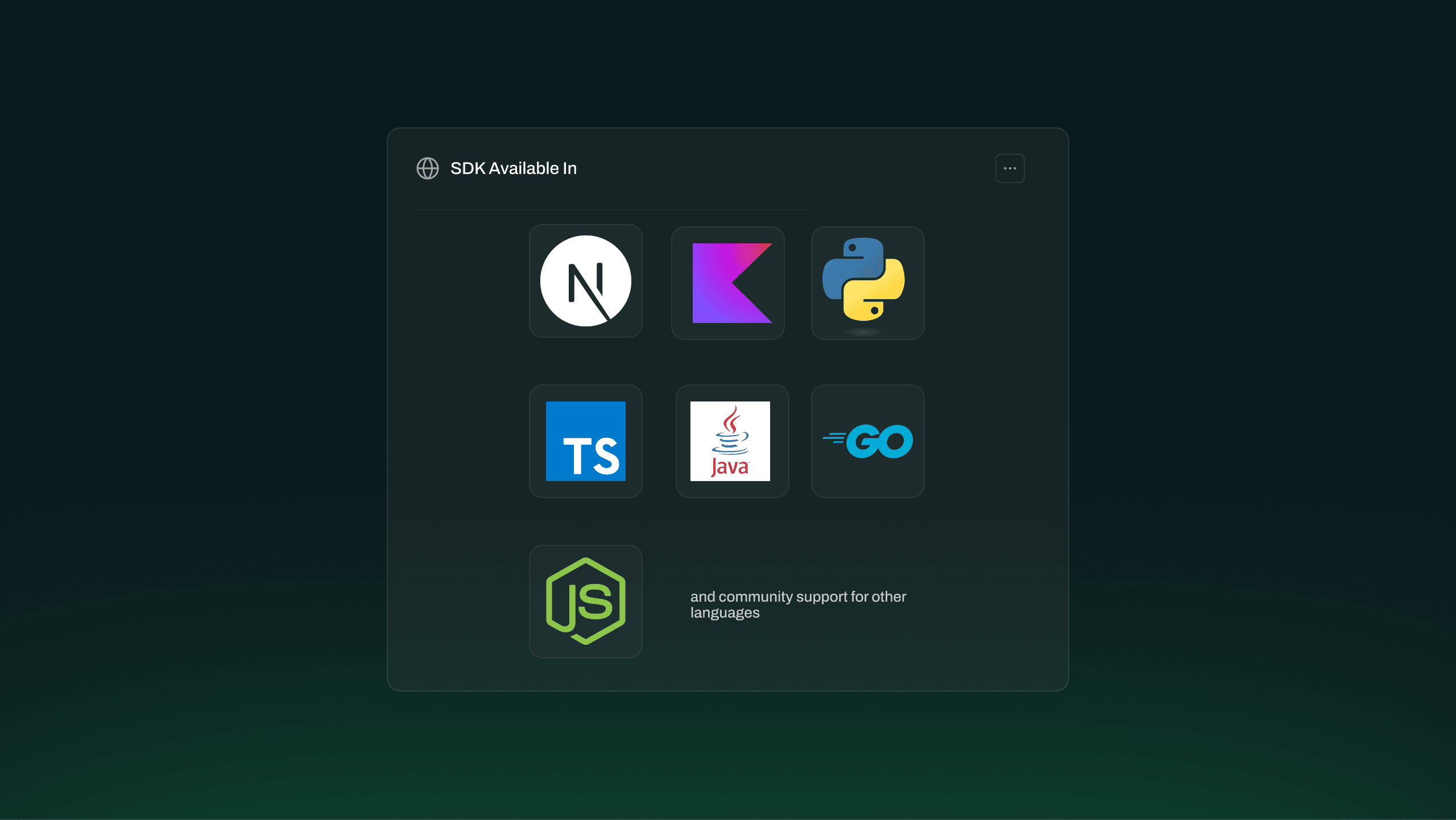
UI
If your API keys are accessible to your users on your dashboard, then you need to implement the necessary UI. This UI will let your customers issue, revoke, rotate, enable, delete keys with ease. Your UI will also ensure that in a multi-tenant environment the right keys are loaded.
At Oneloop, we have built React embeddable components that you can show to your users with a single line of code, allowing your users to do everything.
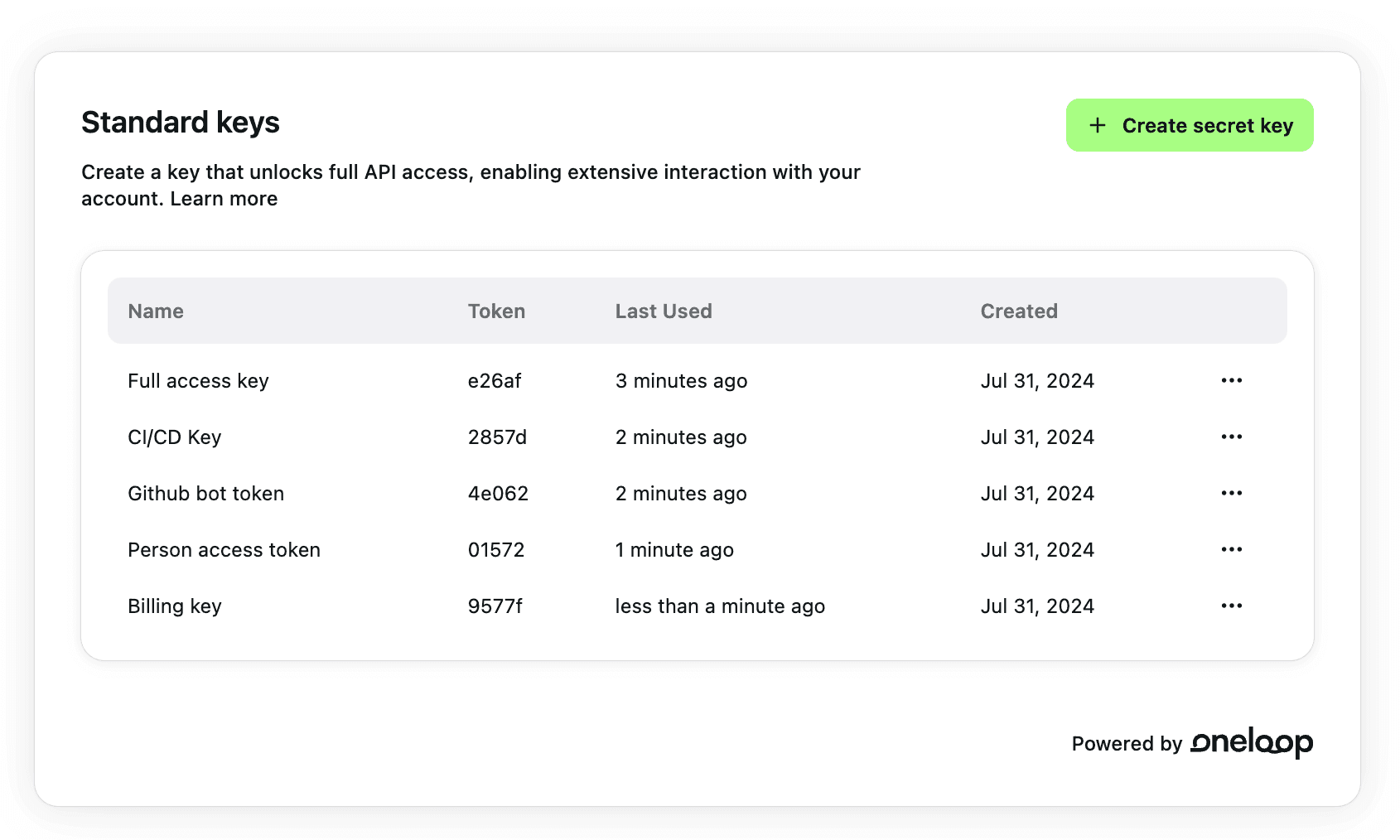
Conclusion
As you can see there is a lot that goes in building a secure, scalable and speedy API key system.
Every company building an API ends up building all these components in-house spending weeks initially and then time on future maintenance and feature-building work. But instead companies should focus on reducing time-to-market, and not building an MVP API Key infrastructure when Oneloop exists.
Sign up here, or schedule a call with me here if you want to get done with keys in 5 mins.